https://programmers.co.kr/learn/courses/30/lessons/64061
프로그래머스
코드 중심의 개발자 채용. 스택 기반의 포지션 매칭. 프로그래머스의 개발자 맞춤형 프로필을 등록하고, 나와 기술 궁합이 잘 맞는 기업들을 매칭 받으세요.
programmers.co.kr
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
|
#include <string>
#include <vector>
#include <stack>
using namespace std;
int solution(vector<vector<int>> board, vector<int> moves) {
int answer = 0;
stack<int> st;
//열마다 제일 위에 있는 값의 높이(행)
vector<int> top(board.size(), board.size());
//i는 열 j는 행
for (int i = 0; i < board.size(); i++) {
for (int j = 0; j < board.size(); j++) {
if (board[j][i] != 0) {
top[i] = j;
break;
}
}
}
for (int i = 0; i < moves.size(); i++) {
int col = moves[i]-1;
if (top[col] != board.size()) {
if(st.empty() || st.top() != board[top[col]][col]){ st.push(board[top[col]][col]); }
else {
st.pop();
answer += 2;
}
board[top[col]][col] = 0;
top[col]++;
}
}
return answer;
}
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4ftext-decoration:none">Colored by Color Scripter
|
NxN의 배열의 임의의 열을 하나의 stack 구조로 생각하고 문제를 접근
각 열마다 가장 위 값의 위치 인덱스를 top 벡터에 저장
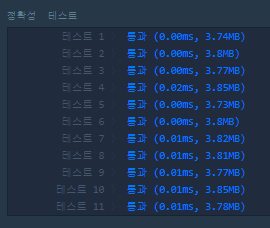
'알고리즘(C++) > 프로그래머스 level1' 카테고리의 다른 글
실패율 - 2019 KAKAO BLIND RECRUITMENT (0) | 2020.04.04 |
---|---|
예산 (0) | 2020.04.04 |
체육복 (0) | 2020.04.04 |
x만큼 간격이 있는 n개의 숫자 (0) | 2020.04.04 |
핸드폰 번호 가리기 (0) | 2020.04.03 |